Python is widely used by many developers all around the world to create mind-blowing applications. What’s more exciting about Python is that it’s dynamically programmed. That means you don’t have to declare variable types when constructing a variable. It’s automatically selected. On top of that, error handling allows you to catch and handle multiple errors of the same type. Incomplete parenthesis, brackets, and quotes all throw different types of errors. EOL While Scanning String Literal Error is one of the errors while parsing strings in Python.
EOL While Scanning String Literal Error appears when your python code ends before ending the string quotes. In simpler terms, your string quotes are missing in the code either by human mistake or incorrect syntax mistake. As Python falls under interpreted language (which means your code is executed line by line without compiling), all the statements before the EOL errors are executed properly.
In this post, we’ll have a look at all the causes and solutions of this error. Moreover, some bonus FAQs to avoid EOL errors in the future.
What exactly is EOL While Scanning String Literal?
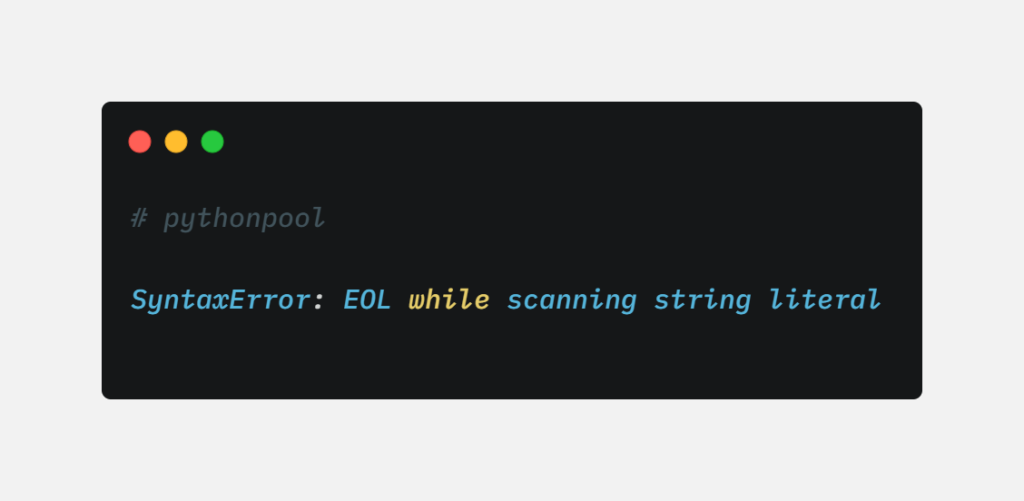
Without a complete grasp of a problem, it’s very difficult to debug it. In layman’s terms, EOL stands for “End Of the Line”. It means that your codes ended without completing a certain syntax end. For example, when you declare a string in Python, quotes (“) are used. At this time, if you don’t end the line with another quote (“), it’ll throw EOL While Scanning Error in the SyntaxError category.
EOL is triggered when your python interpreter has completed reading the last character of your code. In simpler words, it’s called when the file ends. Upon calling the EOL, the interpreter checks if there are incomplete string quotes, brackets, and curly brackets. If yes, then the SyntaxError is thrown.
If you are a beginner, you’ll have a hard time understanding what is string literal. The following section will help you to understand it.
What is String Literal in Python?
String literal is a set of characters enclosed between quotation marks (“). All the characters are noted as a sequence. In Python, you can declare string literals using three types, single quotation marks (‘ ‘), double quotation marks (” “), and triple quotation marks (“”” “””). Strings are arrays and their characters can be accessed by using square brackets. The following example will help you to understand String Literals.
String Literal Example
example1 = 'Single Quotes String'
example2 = "Double Quotes String"
example3 = """Triple Quotes String"""
print(example1[0]) # will print S
Causes of EOL While Scanning String Literal Error
There are known causes for the EOL error in Python. Once you know all of them, you can easily debug your code and fix it. Although, these causes are not necessarily the only known causes for the error. Some other errors might also result in throwing EOL error. Let’s jump right into all the causes –
Cause 1: Unclosed Single Quotes
String Literals in python can be declared by using single quotes in your program. These literals need to be closed within two single quotes sign (‘ ‘). If you failed to enclose the string between these two quotes, it’ll throw EOL While Scanning String Literal Error. Moreover, if you give an extra single quote in your string it’ll also throw the same error. Following examples will help you to understand –
Example 1 –
example1 = 'Single Quotes String
example2 = "Double Quotes String"
example3 = """Triple Quotes String"""
example1[0] # will print S
In this example, there is a missing end single quote in line 1. This missing quote causes the interpreter to parse the next lines as strings that are incorrect. Adding a single quote at the end of line 1 can fix this problem.
Example 2 –
x = 'This is a String
print(x)
In this example, there is a missing single quote at the end of line 1.
Example 3 –
x = 'It's awesome'
print(x)
In this special example, there three single quotes in the first line. According to python, the string for x variable ends at the send single quote. The next part will be treated as a part of another code, not a string. This causes SyntaxError to appear on your screen.
Cause 2: Unclosed Double Quotes
String Literals can also be declared by using double-quotes. In most of hte programming languages, double quotes are the default way to declare a string. So, if you fail to enclose the string with double quotes it’ll throw SyntaxError. Moreover, if you’ve used an odd number of quotes (“) in your string, it’ll also throw this error because of the missing quote. The following example will help you to understand –
Example 1 –
example1 = 'Single Quotes String'
example2 = "Double Quotes String
example3 = """Triple Quotes String"""
example1[0] # will print S
In this example, there is a missing double quote at the end of the second line. This missing quote causes the interpreter to parse all the following codes as part of the string for variable example2. In the end, it throws an EOL error when it reaches the end of the file.
Example 2 –
x = "This is a String
print(x)
Similarly, there is a missing double quote at the end of line 1.
Example 3 –
x = "It"s awesome"
print(x)
In this special example, there three double quotes in the first line. According to python, the string for x variable ends at the send single quote. The next part will be treated as a part of another code, not a string. This causes SyntaxError to appear on your screen.
Cause 3: Unclosed Triple Quotes
In Python, there is a special way of declaring strings using three double quotes (“””). This way is used when you have to include double quotes and single quotes in your string. With this type of declaration, you can include any character in the string. So, if you did not close this triple quotes, I’ll throw an EOL error. Following examples will help you to understand –
Example 1 –
In line 3 of the example, there is a missing quote from the set of triple quotes. As a result, the python interpreter will treat all the following lines as a part of the string for example3 variable. In the end, as there are no ending triple quotes, it’ll throw EOL error.
example1 = 'Single Quotes String'
example2 = "Double Quotes String"
example3 = """Triple Quotes String""
example1[0] # will print S
Example 2 –
Similar to example 1, two of the quotes are missing from line 1.
x = """This is a String"
print(x)
Cause 4: Odd Number of Backslashes in Raw String
Backslashes are used in the string to include special characters in the string. For example, if you want to add double quotes in a doubly quoted string, you can use \” to add it. Every character after backslashes has a different meaning for python. So, if you fail to provide a corresponding following character after the backslash (\), you’ll get EOL While Scanning String Literal Error. Following examples will help you understand it –
Example 1 –
The following string is invalid because there is no following character after the backslash. Currently, python processes the strings in the same way as Standard C. To avoid this error put “r” or “R” before your string.
x = "\"
Example 2 –
The following example contains an odd number of backslashes without the following characters. This causes the EOL error to raise as the interpretation expects the next character.
x = "\\\"
Example 3 –
The last backslash in the string doesn’t have the following character. This causes the compiler to throw an error.
x = "\\\"\"
How do you fix EOL While Scanning String Literal Error?
Since the causes of the errors are clear, we can move on to the solution for each of these errors. Although each cause has a different way of solving it, all of them belong to string literals. All of these errors are based on incomplete string literals which are not closed till the end. Following solutions will help you to solve each of these errors –
Solution for Single Quoted Strings
Fixing incomplete quoted strings is an easy task in Python. Firstly, look for the string declarations in your code. After finding it, check if the string has incomplete end single quotes (‘). Also, check if the string has any extra single quotes. After locating the string, add a single quote at the end of the string.
Example 1 –
example1 = 'Single Quotes String' # added single quote here
example2 = "Double Quotes String"
example3 = """Triple Quotes String"""
example1[0] # will print S
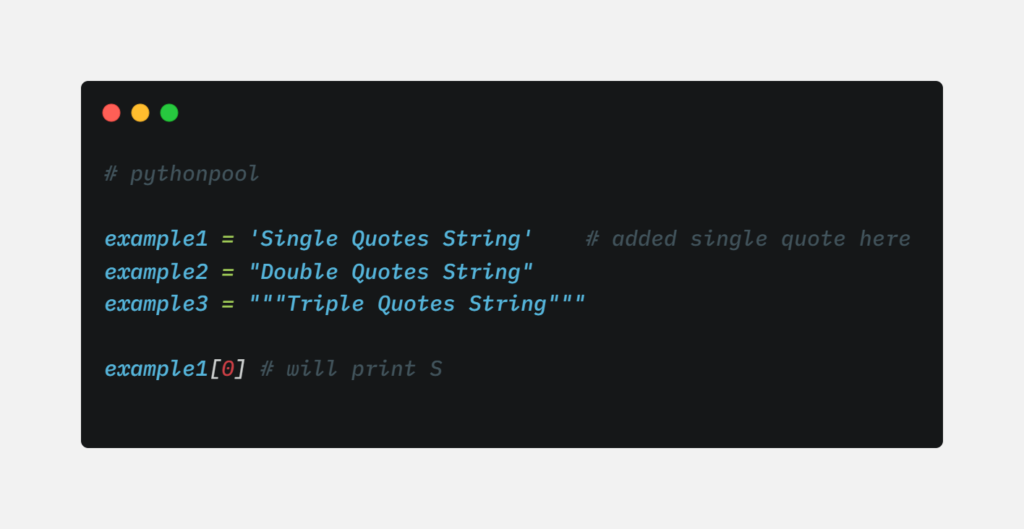
Solution for double quoted Strings in EOL While Scanning String Literal
Similarly, for double quotes, check if any of the string declarations in your code have missing double quotes (“). If not, check for an additional double quote inside the string. You can use the Find option in your IDE to find it quickly. Locate this error and fix it by adding an extra double quote at the end of this line.
Example –
example1 = 'Single Quotes String'
example2 = "Double Quotes String" # added double quote here
example3 = """Triple Quotes String"""
example1[0] # will print S
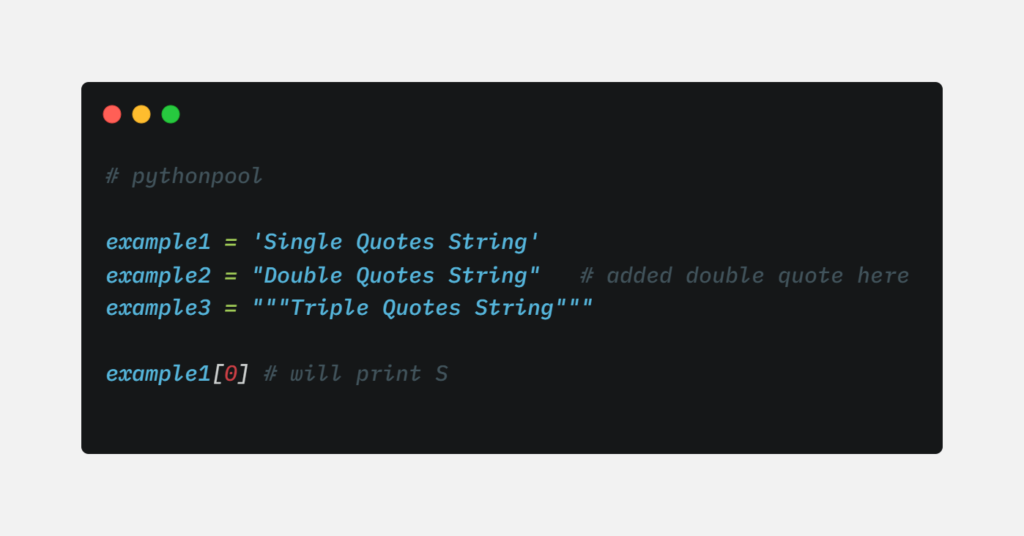
Solution for incomplete backslashes in Strings
Firstly, check if your codes contains and backslashes. If yes, move to the specific line and check if it’s included inside the string. Currently, the backslashes allow us to add special characters in the string. If your string contains such backslashes, remove them and try to run the code. Additionally, if you want to add special characters, you can use the tripe quoted strings as well. Following examples can help you to understand it properly –
# invalid
x = "\"
x = "\\\"
x = "\\\"\"
# valid
x = "\\"
x = "\\\\"
x = "\\\""
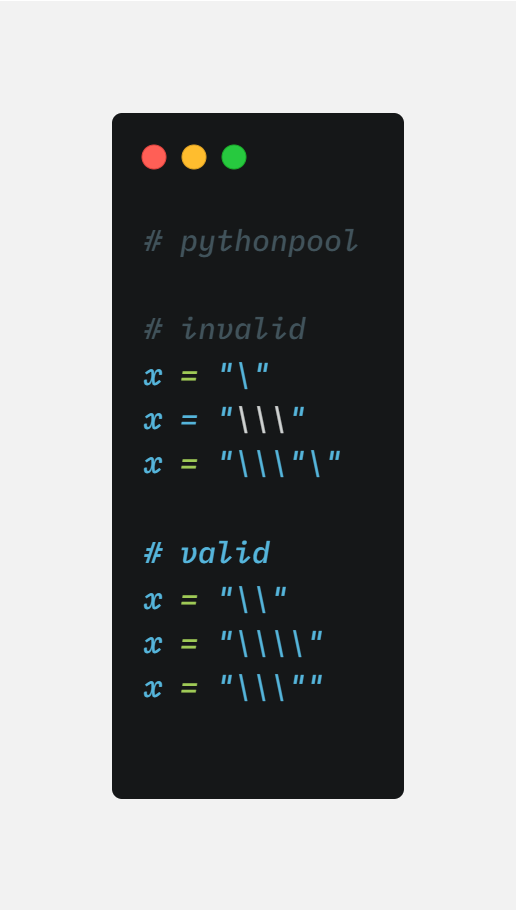
To add special characters in the string, you can use backslashes in the string. For example, \t adds a new tab space, \n adds a new line, \\ adds a literal backslash, and many others. Make sure that you use them carefully to avoid any EOF While Scanning Errors.
EOL While Scanning Triple Quoted String Literal Error
Triple Quoted Strings are a powerful way of defining strings in Python. It can be used if you want to add characters like double quotes, single quotes, and other special characters in the string. Multiline Line String Literals can also be declared by using Tripe Quoted Strings. Also, the documentation of codes is done via triple quotes in python. Many times, we mistakenly use less than three quotes to initialize it. In such cases, EOL while scanning triple quoted string literal error appears.
The basic syntax of triple quoted string is –
x = """This is a triple quoted string""""
Using the above method, you can declare strings in triple quotes. Check – How to Remove Quotes From a String in Python
Coming back to our error, whenever a user uses less than 6 quotes (3 at front and 3 at the end), the interpreter throws an EOL SyntaxError. To fix this, make sure that you keep the proper 3 + 3 quotes structure in your code as shown above. Follow this example to get an idea –
# invalid
x = """Incorrect String""
x = ""Incorrect String"""
x = "Incorrect String"""
x = """Incorrect String"
x = """Incorrect "String""
# valid
x = """Correct String"""
x = """Correct "String"""
x = """Correct ""String"""
Note – You can add any number of single and double quotes inside the string but the three quotes on start and end are mandatory.
How to fix SyntaxError unexpected EOF while parsing?
As EOL While Scanning String Literal Error occurs due to incomplete strings, unexpected EOF while parsing occurs when there are other incomplete blocks in the code. The interpreter was waiting for certain brackets to be completed but they never did in the code. The main cause of this error is incomplete brackets, square brackets, and no indented blocks.
For example consider a, “for” loop in your code that has no intended block following it. In such cases, the Syntax Error EOF will be generated.
Moreover, check the parameters passed in your functions to avoid getting this error. Passing an invalid argument can also result in this error. Following code, an example can help you to understand the parsing errors –
Example 1 –
In this example, there is a bracket missing from the print() statement. The interpreter reaches the End of the Line before the bracket completes, resulting in SyntaxError. Such errors can be solved by simply adding another bracket.
Error –
x = "Python Pool is awesome"
print(x.upper() # 1 bracket is missing
Solution –
x = "Python Pool is awesome"
print(x.upper())
Example 2 –
In this example, there is no code block after the “for” statement. The python interpreter expects an indented code block after the for a statement to execute it, but here it’s not present. As a result, this code throws an EOF error. To solve this error, insert an intended block at the end.
Error –
x = 5
for i in range(5):
Solution –
x = 5
for i in range(5):
print(x)
Common FAQs
Following are some of the common FAQs related to this topic –
How to use \n in Python?
\n refers to a new line in programming languages. To use it in python, you need to declare a string by using double quotes (“) and then add a “\n” inside it to create a new line. Following is an example for it –
x = "First line\nSecond Line"
print(x)
Output –
First line
Second Line
How to Use Backslash in Python?
Backslashes are a great way to add special characters to your string. It is used to add quotes, slashes, new lines, tab space, and other characters in the string. To use it, you first need to declare a string using double quotes and then use “\” to add characters.
x = "This adds a tab space\tbetween"
print(x)
Output –
This adds a tab space between
Which form of string literal ignores backslashes?
Only raw strings literals ignore the backslashes in the Python code. To declare a raw string, you have to mention an “r” or “R” before your string. This helps to understand the python interpreter to avoid escape sequences,
x = r'\pythonpool'
print(x)
Output –
\pythonpool
Must Read
Python getopt Module: A – Z Guide
4 Ways to Draw a Rectangle in Matplotlib
5 Ways to Check if the NumPy Array is Empty
7 Powerful ways to Convert string to list in Python
Final Words: EOL While Scanning String Literal
Strings have helpful in thousands of ways in Python. As using them can provide easy access to a sequence of characters and their attributes. The only problem is that you have to take care of their syntax. Any invalid syntax and invalid backslashes in the string can cause EOF errors to appear. To avoid this error, follow all the steps from the post above.
Happy Coding!
Please Do help me with this code below
from question_model import Question
from data import question_data
from quiz_brain import QuizBrain
question_bank = []
for question in question_data:
question_text = question[“question”]
question_answer = question[“correct_answer”]
new_question = Question(question_text, question_answer)
question_bank.append(new_question)
quiz = QuizBrain(question_bank)
while quiz.still_has_questions():
quiz.next_question()
while input(“Do you want to play a Quiz game.Type ‘y’ for yes and ‘n’ for no: “):
print(f”Your final score was: {quiz.score}/{quiz.question_number)
print(“You have completed the Quiz!”)
You have unclosed double quotes on second last line.